In this tutorial, our focus is on making a User/Member login activity using fragments. With the help of Fragments we will use the same activity to show User login area and also Members Login area.
Prerequisite for this tutorial:
- You should be know how to make an Activity
- And most importantly you should have prior Knowledge of Fragments. For practising basic Fragment implementation refer to Android Simple Fragment Example
We have used only three activities for this:
- MainActivity(which represents the Login Screen)
- Fragments for Members area
- Fragment for New Users
As shown in above image, we have two buttons "Bti Members" and "Others". If you click on any of the button their respective screen will be shown.
Here goes the whole code:
activity_other_fragment.xml
<?xml version="1.0" encoding="utf-8"?><RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.android.fraguserlogin.OtherFragment"> <RelativeLayout android:layout_width="match_parent" android:layout_height="300dp" android:background="#fff"> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText2" android:layout_marginTop="5dp" android:layout_marginBottom="5dp" android:hint="Username/Email id" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText3" android:hint="Password" android:layout_below="@+id/editText2" android:layout_marginBottom="5dp" android:layout_alignParentStart="true" /> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Login" android:id="@+id/button" android:layout_below="@+id/editText3" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="or" android:layout_marginTop="8dp" android:layout_marginBottom="8dp" android:id="@+id/textView3" android:layout_below="@+id/button" android:layout_centerHorizontal="true" /> <ImageView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/imageView" android:background="@drawable/fb" android:layout_below="@+id/textView3" android:layout_centerHorizontal="true" /> </RelativeLayout> </RelativeLayout>
OtherFragment.java
package com.example.android.fraguserlogin; import android.app.Fragment; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class OtherFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { return inflater.inflate(R.layout.activity_other_fragment,container,false); } }
activity_member_area.xml
<?xml version="1.0" encoding="utf-8"?><RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.android.fraguserlogin.Member_Area" android:background="#fff"> <RelativeLayout android:layout_width="match_parent" android:layout_height="300dp" android:background="#fff"> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_marginTop="5dp" android:layout_marginBottom="5dp" android:hint="Membership Number" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Login" android:id="@+id/button" android:layout_marginTop="10dp" android:layout_below="@+id/editText" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="or" android:layout_marginTop="8dp" android:layout_marginBottom="8dp" android:id="@+id/textView3" android:layout_below="@+id/button" android:layout_centerHorizontal="true" /> <ImageView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/imageView" android:background="@drawable/fb" android:layout_below="@+id/textView3" android:layout_centerHorizontal="true" /> </RelativeLayout> </RelativeLayout>
Member_Area.java
package com.example.android.fraguserlogin; import android.app.Fragment; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class Member_Area extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { return inflater.inflate(R.layout.activity_member__area,container,false); } }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="20dp" android:paddingRight="20dp" android:paddingTop="@dimen/activity_vertical_margin" android:background="#7f7fff" android:orientation="vertical" tools:context="com.example.android.fraguserlogin.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="BTI INC." android:textStyle="bold" android:id="@+id/textView" android:layout_gravity="center" android:textColor="#EFFBF8" android:textSize="25sp" android:layout_marginTop="10dp" android:layout_marginBottom="30dp" /> <RelativeLayout android:layout_width="match_parent" android:layout_height="380dp" android:background="#fff" > <ImageButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/mmbr_btn" android:background="@drawable/button_custom" android:layout_alignParentTop="true" android:layout_marginLeft="40dp" android:layout_alignParentStart="true" /> <ImageButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/othr_btn" android:background="@drawable/button_custom2" android:layout_alignParentTop="true" android:layout_toEndOf="@+id/mmbr_btn" /> <RelativeLayout android:id="@+id/fregment_layout" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_centerVertical="true" android:layout_below="@+id/mmbr_btn" android:layout_marginBottom="20dp" android:layout_centerHorizontal="true"> <!-- adding Members Fragment to Main Fragment--> <fragment android:name="com.example.android.fraguserlogin.OtherFragment" android:id="@+id/fragment_container" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout> </RelativeLayout> </LinearLayout>
MainActivity.Java
package com.example.android.fraguserlogin; import android.app.Fragment; import android.app.FragmentManager; import android.app.FragmentTransaction; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.ImageButton; import android.widget.Toast; public class MainActivity extends AppCompatActivity { ImageButton mbr_btn, othr_btn; Fragment fr; FragmentManager fm; FragmentTransaction ft; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mbr_btn = (ImageButton)findViewById(R.id.mmbr_btn); othr_btn = (ImageButton)findViewById(R.id.othr_btn); mbr_btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { fr = new Member_Area(); fm = getFragmentManager(); ft = fm.beginTransaction(); ft.add(R.id.fragment_container, fr); ft.addToBackStack(null); ft.commit(); Toast.makeText(getApplicationContext(),"Members Login Area",Toast.LENGTH_LONG).show(); } }); othr_btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { fr = new OtherFragment(); fm = getFragmentManager(); ft = fm.beginTransaction(); ft.add(R.id.fragment_container, fr); ft.addToBackStack(null); ft.commit(); Toast.makeText(getApplicationContext(),"Login Area for New Users",Toast.LENGTH_LONG).show(); } }); } }
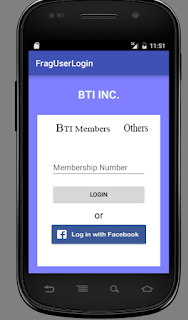
Just want to thanks for this helpful and knowledgeable
ReplyDeleteBest Web Development Company
Best Web Development Company